course on software development using OOP & Design patterns
Practice in OOP and Design Patterns
This course gave me a solid foundation in advanced C, and Object Oriented Programming & Design Patterns in C++, Java, and Python. Below are some key projects from the course.
Projects
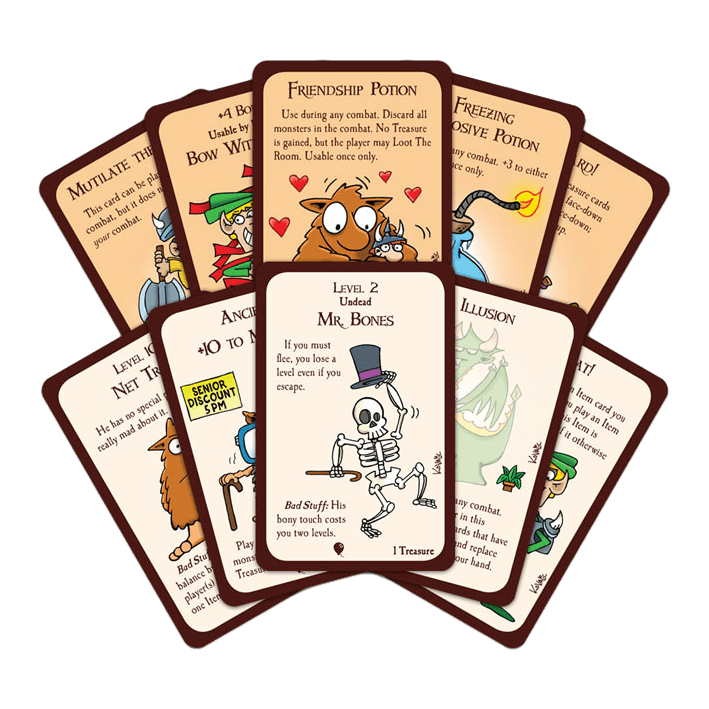
Custom Implementation of Munchkin Card Game (C++)
This project implements a popular card game using object-oriented programming techniques such as inheritance, abstract classes, virtual functions, smart pointers, and exception handling, as well as the Standard Template Library (STL).
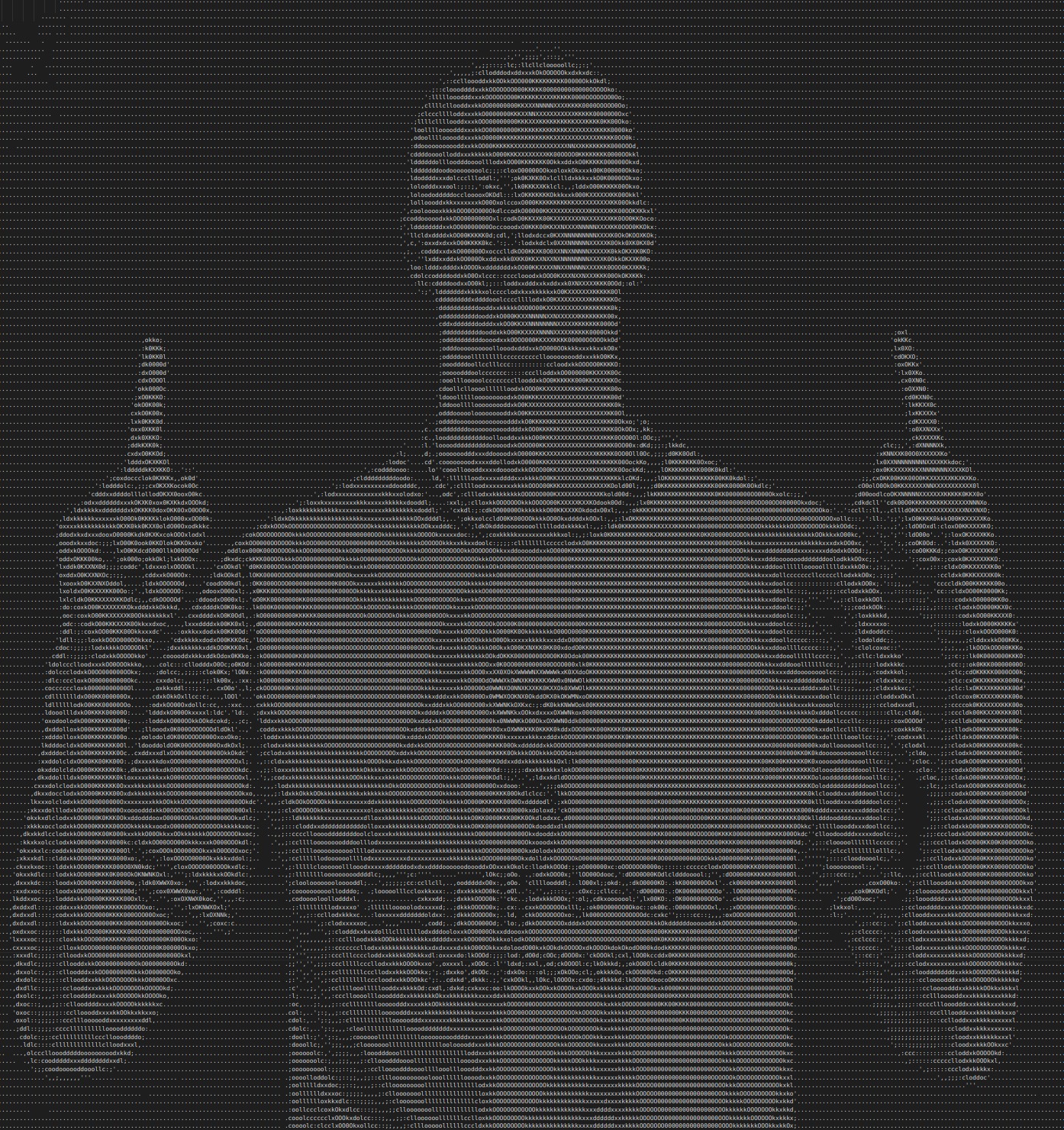
Run-Length Encoding for ASCII Art (C)
Run-length encoding (RLE) is a lossless data compression technique where consecutive data elements with the same value are represented by a single value and its frequency rather than being stored individually. This project, written in C, allows a user to input an ASCII art text file, and a compressed version of the text file is returned.
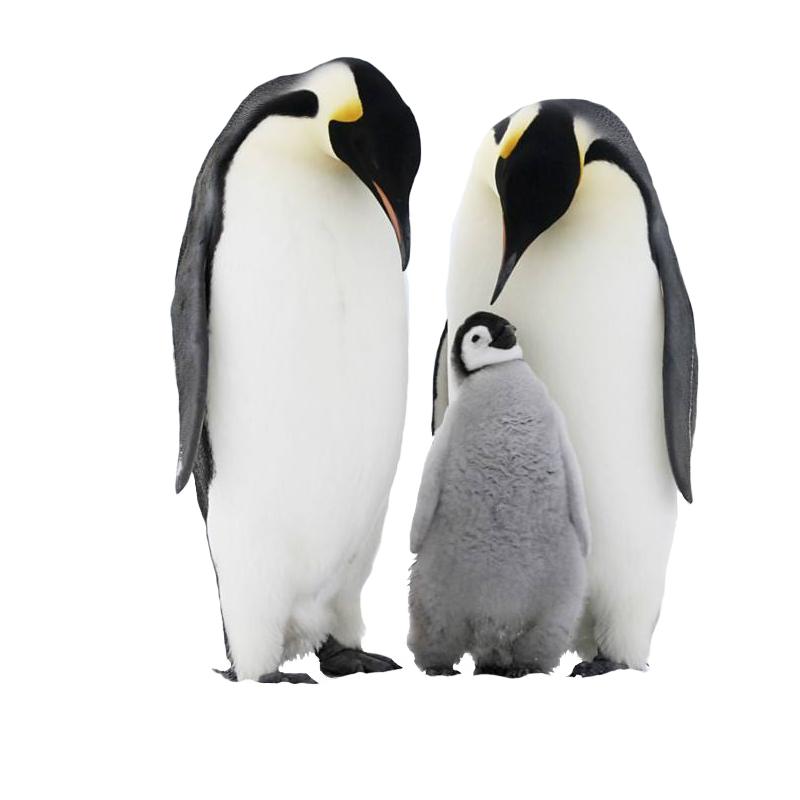
Zoo Planner (Java)
Zooplanner is a system programming project that simulates the management of a zoo. It uses various software design patterns such as Factory, Observer, and Singleton, to achieve this.
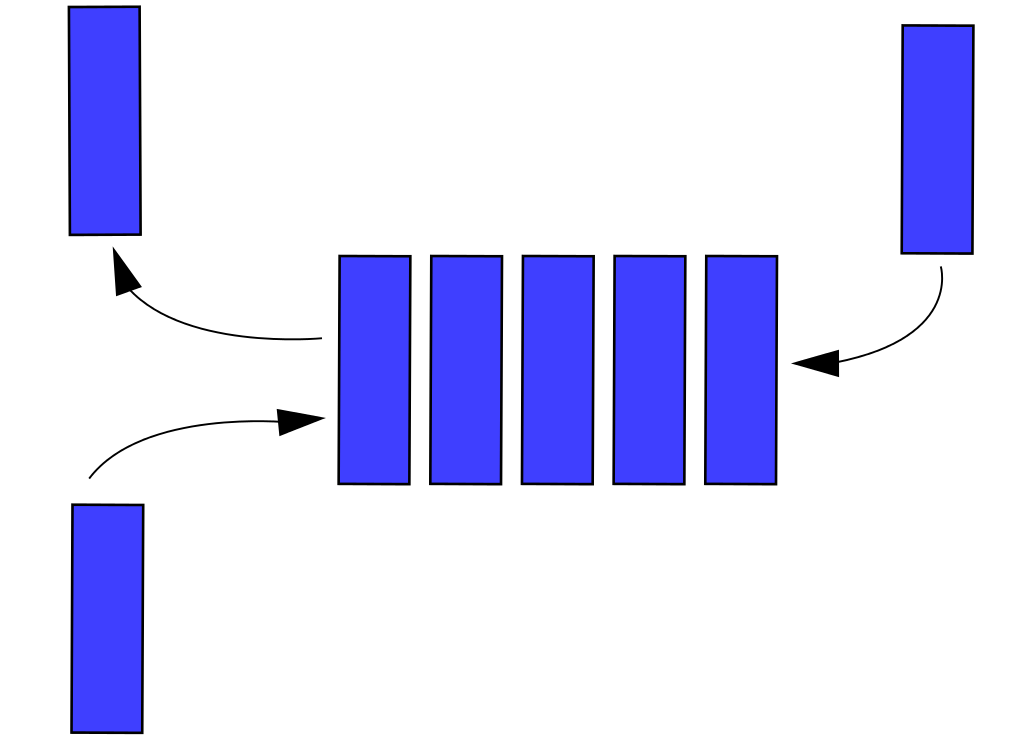
Generic Queue (C++)
This project is an implementation of a generic FIFO queue from scratch, including use of templates, iterators, and operator overloading.
Skills Used/Learned
- Object-Oriented Programming (OOP) concepts such as classes, objects, inheritance, polymorphism, and encapsulation.
- Experience in Advanced C, C++, and Java
- Memory handling (Valgrind)
- Exception handling
- Exposure to Design Patterns (Creational, Structural, Behavioral)